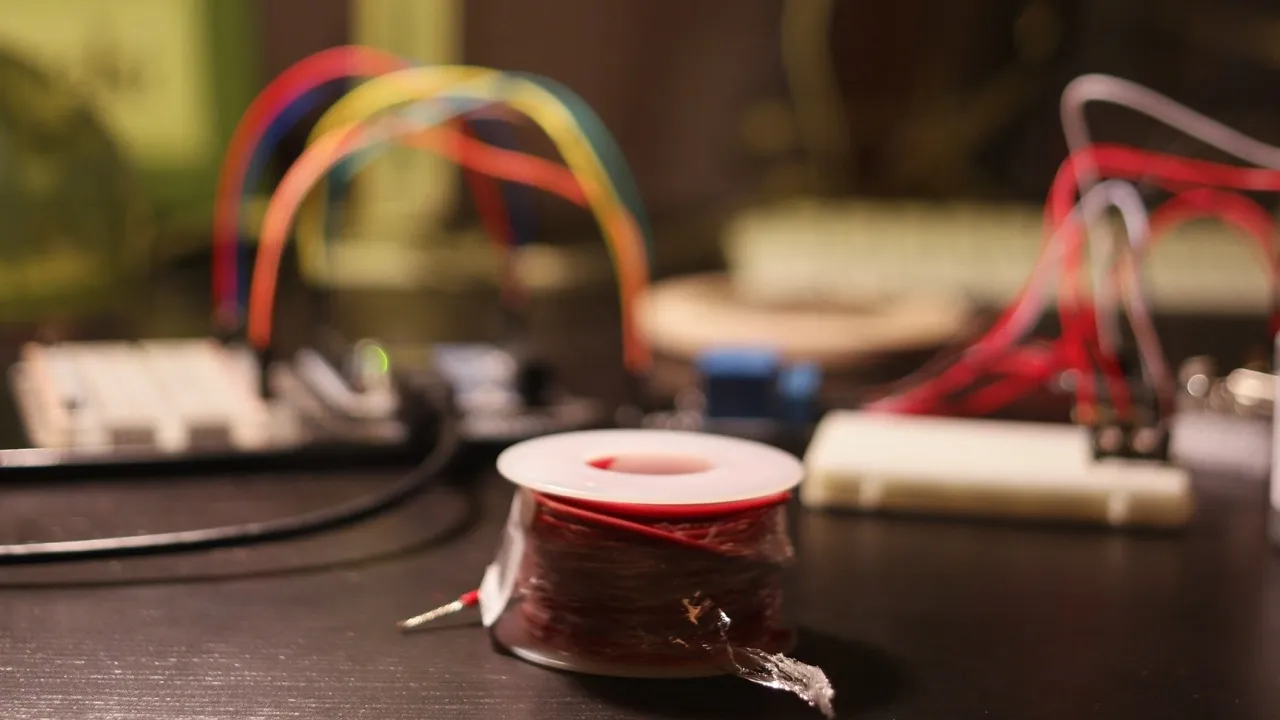
One of the things that really clicked with me when I got into Python way
back in the day was how easy it was to write and run a simple program. Just
start writing code in a coolprogram.py
file, run python coolprogram.py
and
you’re off and running.
Years later when I started doing mobile development with Android, it felt nothing like that. There was a big IDE to learn and all kinds of Java and XML files that were directly involved with getting an app running.
It felt like such a step back from python coolprogram.py
.
coolprogram.py
Python is far from perfect, but it has such a great story for anyone just
getting started with coding. It will run your code in coolprogram.py
from
top to bottom without any ceremony. No imports to do basic things like
input/output, no main
function required, just write and run your code.
for i in range(5):
print("Got", i)
$ python coolprogram.py
Got 0
Got 1
Got 2
Got 3
Got 4
The sky’s the limit from there - you’re free to being learning about imports,
functions, multiple modules, etc. But the barrier to entry for just whetting
your appetite is fantastically low and you can really get quite far with a
single .py
file.
Single File Apps in Flutter
There really isn’t a mobile app equivalent to that, but to be fair, what we expect of a mobile app is much higher than we expect of a script that runs on the command line.
I think the closest you can come today is getting started with a Flutter app.
Once you have Flutter installed1, you can start a new project like:
$ flutter create --empty cool_flutter_app
$ cd cool_flutter_app
Then it’s a matter of opening lib/main.dart
in your favorite editor, making
changes to it and then running flutter run
.
Here’s what something like coolprogram.py
looks like in Flutter:
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: Scaffold(
body: SafeArea(
child: Column(
children: List.generate(
5,
(index) => Text(
'Got $index',
style: const TextStyle(fontSize: 24.0),
),
),
),
),
),
),
);
}
I know what you’re thinking. It’s 20x as long as the simple Python version! But again, mobile apps are by nature more complex than scripts that run in the terminal.
This app handles:
- Text directionality (LtR and RtL)
- Camera notches
- Device rotation
- Custom font rendering
Flutter also gives you the ability to “hot reload” your app with your latest changes, avoiding a new compile/install/launch cycle.
Simplifying
If you just want to try out an idea with Flutter and don’t want to start a new app or even install the framework, give DartPad a try. You can write a “single file” app directly in your browser and interact with it live.
It has a similar feel to playing around with Python code in the interactive interpreter (REPL).
Conclusion
Even though you can write a whole app in a single main.dart
file, there are lots
of other supporting files and of course the whole Flutter framework behind the scenes.
If you squint though, you get a similar experience as you do in Python, where you can start from something relatively small but go bigger as you learn. Also similar to Python, what you create with Flutter can run on a number of platforms (Mac, Windows, Linux, Android, iOS, Web).
If you’re getting into coding and want to try your hand at mobile, or have the ability to see your apps run on multiple platforms, try starting with a single file Flutter app and see where it leads you!
Footnotes
-
I will confess that the installation instructions for developing an iOS app on a Mac are pretty daunting … ↩